That is a different and slightly more flexible calculator, but like the other ones that I had found online it does not generate a round trip of calculations that proves lossless. Here are the 2 flows I created to check this. If you want to look at it, I would love to find that I introduced an error.
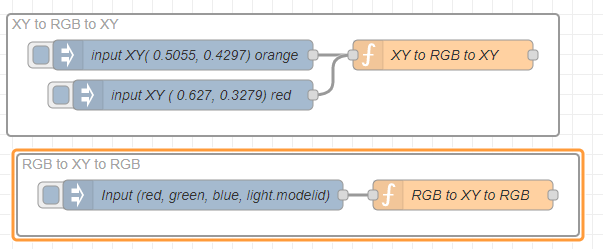
[{"id":"1506189cf70c13eb","type":"group","z":"f1716bd4caacceb3","name":"XY to RGB to XY","style":{"label":true,"fill":"#ffffff"},"nodes":["4992e43182ef4e9a","f0eec26657aa8a73","029be8e19327e7c9"],"x":1474,"y":319,"w":552,"h":122},{"id":"4992e43182ef4e9a","type":"function","z":"f1716bd4caacceb3","g":"1506189cf70c13eb","name":"XY to RGB to XY","func":"class ColorConverter {\n static getGamutRanges() {\n let gamutA = {\n red: [0.704, 0.296],\n green: [0.2151, 0.7106],\n blue: [0.138, 0.08]\n };\n\n let gamutB = {\n red: [0.675, 0.322],\n green: [0.409, 0.518],\n blue: [0.167, 0.04]\n };\n\n let gamutC = {\n red: [0.692, 0.308],\n green: [0.17, 0.7],\n blue: [0.153, 0.048]\n };\n\n let defaultGamut = {\n red: [1.0, 0],\n green: [0.0, 1.0],\n blue: [0.0, 0.0]\n };\n\n return { \"gamutA\": gamutA, \"gamutB\": gamutB, \"gamutC\": gamutC, \"default\": defaultGamut }\n }\n\n static getLightColorGamutRange(modelId = null) {\n let ranges = ColorConverter.getGamutRanges();\n let gamutA = ranges.gamutA;\n let gamutB = ranges.gamutB;\n let gamutC = ranges.gamutC;\n\n let philipsModels = {\n LST001: gamutA,\n LLC010: gamutA,\n LLC011: gamutA,\n LLC012: gamutA,\n LLC006: gamutA,\n LLC005: gamutA,\n LLC007: gamutA,\n LLC014: gamutA,\n LLC013: gamutA,\n\n LCT001: gamutB,\n LCT007: gamutB,\n LCT002: gamutB,\n LCT003: gamutB,\n LLM001: gamutB,\n\n LCT010: gamutC,\n LCT014: gamutC,\n LCT015: gamutC,\n LCT016: gamutC,\n LCT011: gamutC,\n LLC020: gamutC,\n LST002: gamutC,\n LCT012: gamutC,\n };\n\n if (!!philipsModels[modelId]) {\n return philipsModels[modelId];\n }\n\n return ranges.default;\n }\n\n\n static rgbToXy(red, green, blue, modelId = null) {\n function getGammaCorrectedValue(value) {\n return (value > 0.04045) ? Math.pow((value + 0.055) / (1.0 + 0.055), 2.4) : (value / 12.92)\n }\n\n let colorGamut = ColorConverter.getLightColorGamutRange(modelId);\n\n red = parseFloat(red / 255);\n green = parseFloat(green / 255);\n blue = parseFloat(blue / 255);\n\n red = getGammaCorrectedValue(red);\n green = getGammaCorrectedValue(green);\n blue = getGammaCorrectedValue(blue);\n\n let x = red * 0.649926 + green * 0.103455 + blue * 0.197109;\n let y = red * 0.234327 + green * 0.743075 + blue * 0.022598;\n let z = red * 0.0000000 + green * 0.053077 + blue * 1.035763;\n\n let xy = {\n x: x / (x + y + z),\n y: y / (x + y + z)\n };\n\n if (!ColorConverter.xyIsInGamutRange(xy, colorGamut)) {\n xy = ColorConverter.getClosestColor(xy, colorGamut);\n }\n\n return xy;\n }\n\n static xyIsInGamutRange(xy, gamut) {\n gamut = gamut || ColorConverter.getGamutRanges().gamutC;\n if (Array.isArray(xy)) {\n xy = {\n x: xy[0],\n y: xy[1]\n };\n }\n\n let v0 = [gamut.blue[0] - gamut.red[0], gamut.blue[1] - gamut.red[1]];\n let v1 = [gamut.green[0] - gamut.red[0], gamut.green[1] - gamut.red[1]];\n let v2 = [xy.x - gamut.red[0], xy.y - gamut.red[1]];\n\n let dot00 = (v0[0] * v0[0]) + (v0[1] * v0[1]);\n let dot01 = (v0[0] * v1[0]) + (v0[1] * v1[1]);\n let dot02 = (v0[0] * v2[0]) + (v0[1] * v2[1]);\n let dot11 = (v1[0] * v1[0]) + (v1[1] * v1[1]);\n let dot12 = (v1[0] * v2[0]) + (v1[1] * v2[1]);\n\n let invDenom = 1 / (dot00 * dot11 - dot01 * dot01);\n\n let u = (dot11 * dot02 - dot01 * dot12) * invDenom;\n let v = (dot00 * dot12 - dot01 * dot02) * invDenom;\n\n return ((u >= 0) && (v >= 0) && (u + v < 1));\n }\n\n static getClosestColor(xy, gamut) {\n function getLineDistance(pointA, pointB) {\n return Math.hypot(pointB.x - pointA.x, pointB.y - pointA.y);\n }\n\n function getClosestPoint(xy, pointA, pointB) {\n let xy2a = [xy.x - pointA.x, xy.y - pointA.y];\n let a2b = [pointB.x - pointA.x, pointB.y - pointA.y];\n let a2bSqr = Math.pow(a2b[0], 2) + Math.pow(a2b[1], 2);\n let xy2a_dot_a2b = xy2a[0] * a2b[0] + xy2a[1] * a2b[1];\n let t = xy2a_dot_a2b / a2bSqr;\n\n return {\n x: pointA.x + a2b[0] * t,\n y: pointA.y + a2b[1] * t\n }\n }\n\n let greenBlue = {\n a: {\n x: gamut.green[0],\n y: gamut.green[1]\n },\n b: {\n x: gamut.blue[0],\n y: gamut.blue[1]\n }\n };\n\n let greenRed = {\n a: {\n x: gamut.green[0],\n y: gamut.green[1]\n },\n b: {\n x: gamut.red[0],\n y: gamut.red[1]\n }\n };\n\n let blueRed = {\n a: {\n x: gamut.red[0],\n y: gamut.red[1]\n },\n b: {\n x: gamut.blue[0],\n y: gamut.blue[1]\n }\n };\n\n let closestColorPoints = {\n greenBlue: getClosestPoint(xy, greenBlue.a, greenBlue.b),\n greenRed: getClosestPoint(xy, greenRed.a, greenRed.b),\n blueRed: getClosestPoint(xy, blueRed.a, blueRed.b)\n };\n\n let distance = {\n greenBlue: getLineDistance(xy, closestColorPoints.greenBlue),\n greenRed: getLineDistance(xy, closestColorPoints.greenRed),\n blueRed: getLineDistance(xy, closestColorPoints.blueRed)\n };\n\n let closestDistance;\n let closestColor;\n for (let i in distance) {\n if (distance.hasOwnProperty(i)) {\n if (!closestDistance) {\n closestDistance = distance[i];\n closestColor = i;\n }\n\n if (closestDistance > distance[i]) {\n closestDistance = distance[i];\n closestColor = i;\n }\n }\n\n }\n return closestColorPoints[closestColor];\n }\n\n static xyBriToRgb(x, y, bri) {\n function getReversedGammaCorrectedValue(value) {\n return value <= 0.0031308 ? 12.92 * value : (1.0 + 0.055) * Math.pow(value, (1.0 / 2.4)) - 0.055;\n }\n\n let xy = {\n x: x,\n y: y\n };\n\n let z = 1.0 - xy.x - xy.y;\n let Y = bri / 255;\n let X = (Y / xy.y) * xy.x;\n let Z = (Y / xy.y) * z;\n let r = X * 1.656492 - Y * 0.354851 - Z * 0.255038;\n let g = -X * 0.707196 + Y * 1.655397 + Z * 0.036152;\n let b = X * 0.051713 - Y * 0.121364 + Z * 1.011530;\n\n r = getReversedGammaCorrectedValue(r);\n g = getReversedGammaCorrectedValue(g);\n b = getReversedGammaCorrectedValue(b);\n\n let red = parseInt(r * 255) > 255 ? 255 : parseInt(r * 255);\n let green = parseInt(g * 255) > 255 ? 255 : parseInt(g * 255);\n let blue = parseInt(b * 255) > 255 ? 255 : parseInt(b * 255);\n\n red = Math.abs(red);\n green = Math.abs(green);\n blue = Math.abs(blue);\n\n return { r: red, g: green, b: blue };\n }\n}\n\nlet rgb\nlet xy\nlet distances =[]\nnode.warn(\"Gamut A\");\nxy = { x: parseFloat(msg.x), y: parseFloat(msg.y) }\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n xy = ColorConverter.rgbToXy(rgb.r, rgb.g, rgb.b, msg.modelidA);\n xy.x = parseFloat(xy.x.toFixed(4));\n xy.y = parseFloat(xy.y.toFixed(4));\n distances.push(Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y),2),.5));\n if (0.00622 >= Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5)) {\n node.warn({ d:Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y),2),.5),\n \"Original X\": msg.x,\n \"Original Y\": msg.y,\n x: xy.x,\n y: xy.y,\n i: index,\n \"in between r\": rgb.r,\n \"in between g\": rgb.g,\n \"in between b\": rgb.b,\n id: msg.modelidA });\n }\n}\nnode.warn(\"Gamut B\");\nxy = { x: parseFloat(msg.x), y: parseFloat(msg.y) }\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n xy = ColorConverter.rgbToXy(rgb.r, rgb.g, rgb.b, msg.modelidB);\n xy.x = parseFloat(xy.x.toFixed(4));\n xy.y = parseFloat(xy.y.toFixed(4));\n distances.push(Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5));\n if (0.00622 >= Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5)) {\n node.warn({\n d: Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5),\n \"Original X\": msg.x,\n \"Original Y\": msg.y,\n x: xy.x,\n y: xy.y,\n \"in between r\": rgb.r,\n \"in between g\": rgb.g,\n \"in between b\": rgb.b,\n id: msg.modelidA\n });\n }\n}\nnode.warn(\"Gamut C\");\nxy = { x: parseFloat(msg.x), y: parseFloat(msg.y) }\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n xy = ColorConverter.rgbToXy(rgb.r, rgb.g, rgb.b, msg.modelidC);\n xy.x = parseFloat(xy.x.toFixed(4));\n xy.y = parseFloat(xy.y.toFixed(4));\n distances.push(Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5));\n if (0.00622 >= Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5)) {\n node.warn({\n d: Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5),\n \"Original X\": msg.x,\n \"Original Y\": msg.y,\n x: xy.x,\n y: xy.y,\n \"in between r\": rgb.r,\n \"in between g\": rgb.g,\n \"in between b\": rgb.b,\n id: msg.modelidA\n });\n }\n}\nnode.warn(\"Gamut D\");\nxy = { x: parseFloat(msg.x), y: parseFloat(msg.y) }\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n xy = ColorConverter.rgbToXy(rgb.r, rgb.g, rgb.b, null);\n xy.x = parseFloat(xy.x.toFixed(4));\n xy.y = parseFloat(xy.y.toFixed(4));\n distances.push(Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5));\n if (0.00622 >= Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5)) {\n node.warn({\n d: Math.pow(Math.pow(xy.x - parseFloat(msg.x), 2) + Math.pow(xy.y - parseFloat(msg.y), 2), .5),\n \"Original X\": msg.x,\n \"Original Y\": msg.y,\n x: xy.x,\n y: xy.y,\n \"in between r\": rgb.r,\n \"in between g\": rgb.g,\n \"in between b\": rgb.b,\n id: msg.modelidA\n });\n }\n}\n node.warn(Math.min(...distances));\n\nreturn msg","outputs":1,"noerr":0,"initialize":"","finalize":"","libs":[],"x":1910,"y":360,"wires":[[]]},{"id":"f0eec26657aa8a73","type":"inject","z":"f1716bd4caacceb3","g":"1506189cf70c13eb","name":"input XY( 0.5055, 0.4297) orange","props":[{"p":"payload"},{"p":"x","v":" 0.5055","vt":"str"},{"p":"y","v":"0.4297","vt":"str"},{"p":"modelidA","v":"LST001","vt":"str"},{"p":"modelidB","v":"LCT007","vt":"str"},{"p":"modelidC","v":"LCT016","vt":"str"}],"repeat":"","crontab":"","once":false,"onceDelay":0.1,"topic":"","payloadType":"date","x":1650,"y":360,"wires":[["4992e43182ef4e9a"]]},{"id":"029be8e19327e7c9","type":"inject","z":"f1716bd4caacceb3","g":"1506189cf70c13eb","name":"input XY ( 0.627, 0.3279) red","props":[{"p":"payload"},{"p":"x","v":"0.627","vt":"str"},{"p":"y","v":"0.3279","vt":"str"},{"p":"modelidA","v":"LST001","vt":"str"},{"p":"modelidB","v":"LCT007","vt":"str"},{"p":"modelidC","v":"LCT016","vt":"str"}],"repeat":"","crontab":"","once":false,"onceDelay":0.1,"topic":"","payloadType":"date","x":1660,"y":400,"wires":[["4992e43182ef4e9a"]]},{"id":"e66acae9d3caa61c","type":"group","z":"f1716bd4caacceb3","name":"RGB to XY to RGB","style":{"label":true,"fill":"#ffffff"},"nodes":["8c436eb521dba370","b65a023af1a2f2b8"],"x":1484,"y":459,"w":562,"h":82},{"id":"8c436eb521dba370","type":"function","z":"f1716bd4caacceb3","g":"e66acae9d3caa61c","name":"RGB to XY to RGB","func":"class ColorConverter {\n static getGamutRanges() {\n let gamutA = {\n red: [0.704, 0.296],\n green: [0.2151, 0.7106],\n blue: [0.138, 0.08]\n };\n\n let gamutB = {\n red: [0.675, 0.322],\n green: [0.409, 0.518],\n blue: [0.167, 0.04]\n };\n\n let gamutC = {\n red: [0.692, 0.308],\n green: [0.17, 0.7],\n blue: [0.153, 0.048]\n };\n\n let defaultGamut = {\n red: [1.0, 0],\n green: [0.0, 1.0],\n blue: [0.0, 0.0]\n };\n\n return { \"gamutA\": gamutA, \"gamutB\": gamutB, \"gamutC\": gamutC, \"default\": defaultGamut }\n }\n\n static getLightColorGamutRange(modelId = null) {\n let ranges = ColorConverter.getGamutRanges();\n let gamutA = ranges.gamutA;\n let gamutB = ranges.gamutB;\n let gamutC = ranges.gamutC;\n\n let philipsModels = {\n LST001: gamutA,\n LLC010: gamutA,\n LLC011: gamutA,\n LLC012: gamutA,\n LLC006: gamutA,\n LLC005: gamutA,\n LLC007: gamutA,\n LLC014: gamutA,\n LLC013: gamutA,\n\n LCT001: gamutB,\n LCT007: gamutB,\n LCT002: gamutB,\n LCT003: gamutB,\n LLM001: gamutB,\n\n LCT010: gamutC,\n LCT014: gamutC,\n LCT015: gamutC,\n LCT016: gamutC,\n LCT011: gamutC,\n LLC020: gamutC,\n LST002: gamutC,\n LCT012: gamutC,\n };\n\n if (!!philipsModels[modelId]) {\n return philipsModels[modelId];\n }\n\n return ranges.default;\n }\n\n\n static rgbToXy(red, green, blue, modelId = null) {\n function getGammaCorrectedValue(value) {\n return (value > 0.04045) ? Math.pow((value + 0.055) / (1.0 + 0.055), 2.4) : (value / 12.92)\n }\n\n let colorGamut = ColorConverter.getLightColorGamutRange(modelId);\n\n red = parseFloat(red / 255);\n green = parseFloat(green / 255);\n blue = parseFloat(blue / 255);\n\n red = getGammaCorrectedValue(red);\n green = getGammaCorrectedValue(green);\n blue = getGammaCorrectedValue(blue);\n\n let x = red * 0.649926 + green * 0.103455 + blue * 0.197109;\n let y = red * 0.234327 + green * 0.743075 + blue * 0.022598;\n let z = red * 0.0000000 + green * 0.053077 + blue * 1.035763;\n\n let xy = {\n x: x / (x + y + z),\n y: y / (x + y + z)\n };\n\n if (!ColorConverter.xyIsInGamutRange(xy, colorGamut)) {\n xy = ColorConverter.getClosestColor(xy, colorGamut);\n }\n\n return xy;\n }\n\n static xyIsInGamutRange(xy, gamut) {\n gamut = gamut || ColorConverter.getGamutRanges().gamutC;\n if (Array.isArray(xy)) {\n xy = {\n x: xy[0],\n y: xy[1]\n };\n }\n\n let v0 = [gamut.blue[0] - gamut.red[0], gamut.blue[1] - gamut.red[1]];\n let v1 = [gamut.green[0] - gamut.red[0], gamut.green[1] - gamut.red[1]];\n let v2 = [xy.x - gamut.red[0], xy.y - gamut.red[1]];\n\n let dot00 = (v0[0] * v0[0]) + (v0[1] * v0[1]);\n let dot01 = (v0[0] * v1[0]) + (v0[1] * v1[1]);\n let dot02 = (v0[0] * v2[0]) + (v0[1] * v2[1]);\n let dot11 = (v1[0] * v1[0]) + (v1[1] * v1[1]);\n let dot12 = (v1[0] * v2[0]) + (v1[1] * v2[1]);\n\n let invDenom = 1 / (dot00 * dot11 - dot01 * dot01);\n\n let u = (dot11 * dot02 - dot01 * dot12) * invDenom;\n let v = (dot00 * dot12 - dot01 * dot02) * invDenom;\n\n return ((u >= 0) && (v >= 0) && (u + v < 1));\n }\n\n static getClosestColor(xy, gamut) {\n function getLineDistance(pointA, pointB) {\n return Math.hypot(pointB.x - pointA.x, pointB.y - pointA.y);\n }\n\n function getClosestPoint(xy, pointA, pointB) {\n let xy2a = [xy.x - pointA.x, xy.y - pointA.y];\n let a2b = [pointB.x - pointA.x, pointB.y - pointA.y];\n let a2bSqr = Math.pow(a2b[0], 2) + Math.pow(a2b[1], 2);\n let xy2a_dot_a2b = xy2a[0] * a2b[0] + xy2a[1] * a2b[1];\n let t = xy2a_dot_a2b / a2bSqr;\n\n return {\n x: pointA.x + a2b[0] * t,\n y: pointA.y + a2b[1] * t\n }\n }\n\n let greenBlue = {\n a: {\n x: gamut.green[0],\n y: gamut.green[1]\n },\n b: {\n x: gamut.blue[0],\n y: gamut.blue[1]\n }\n };\n\n let greenRed = {\n a: {\n x: gamut.green[0],\n y: gamut.green[1]\n },\n b: {\n x: gamut.red[0],\n y: gamut.red[1]\n }\n };\n\n let blueRed = {\n a: {\n x: gamut.red[0],\n y: gamut.red[1]\n },\n b: {\n x: gamut.blue[0],\n y: gamut.blue[1]\n }\n };\n\n let closestColorPoints = {\n greenBlue: getClosestPoint(xy, greenBlue.a, greenBlue.b),\n greenRed: getClosestPoint(xy, greenRed.a, greenRed.b),\n blueRed: getClosestPoint(xy, blueRed.a, blueRed.b)\n };\n\n let distance = {\n greenBlue: getLineDistance(xy, closestColorPoints.greenBlue),\n greenRed: getLineDistance(xy, closestColorPoints.greenRed),\n blueRed: getLineDistance(xy, closestColorPoints.blueRed)\n };\n\n let closestDistance;\n let closestColor;\n for (let i in distance) {\n if (distance.hasOwnProperty(i)) {\n if (!closestDistance) {\n closestDistance = distance[i];\n closestColor = i;\n }\n\n if (closestDistance > distance[i]) {\n closestDistance = distance[i];\n closestColor = i;\n }\n }\n\n }\n return closestColorPoints[closestColor];\n }\n\n static xyBriToRgb(x, y, bri) {\n function getReversedGammaCorrectedValue(value) {\n return value <= 0.0031308 ? 12.92 * value : (1.0 + 0.055) * Math.pow(value, (1.0 / 2.4)) - 0.055;\n }\n\n let xy = {\n x: x,\n y: y\n };\n\n let z = 1.0 - xy.x - xy.y;\n let Y = bri / 255;\n let X = (Y / xy.y) * xy.x;\n let Z = (Y / xy.y) * z;\n let r = X * 1.656492 - Y * 0.354851 - Z * 0.255038;\n let g = -X * 0.707196 + Y * 1.655397 + Z * 0.036152;\n let b = X * 0.051713 - Y * 0.121364 + Z * 1.011530;\n\n r = getReversedGammaCorrectedValue(r);\n g = getReversedGammaCorrectedValue(g);\n b = getReversedGammaCorrectedValue(b);\n\n let red = parseInt(r * 255) > 255 ? 255 : parseInt(r * 255);\n let green = parseInt(g * 255) > 255 ? 255 : parseInt(g * 255);\n let blue = parseInt(b * 255) > 255 ? 255 : parseInt(b * 255);\n\n red = Math.abs(red);\n green = Math.abs(green);\n blue = Math.abs(blue);\n\n return { r: red, g: green, b: blue };\n }\n}\n// let xy = ColorConverter.rgbToXy(red, green, blue, light.modelid);\n// let xy = ColorConverter.rgbToXy(red, green, blue);\n// let rgb = ColorConverter.xyBriToRgb(x, y, brightness);\n\nnode.warn(\"RGB input \" + msg.red + \" \" + msg.green + \" \" + msg.blue + \" \");\nlet rgb\nnode.warn(\"Gamut A XY result\");\nlet xy = ColorConverter.rgbToXy(msg.red, msg.green, msg.blue, msg.modelidA);\nnode.warn(xy);\nnode.warn(\"RGB output if the RGB matches red and green\");\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n if ((msg.red == rgb.r)&&(msg.green == rgb.g)) {\n node.warn(rgb);\n }\n}\nnode.warn(\"Gamut B XY result\");\nxy = ColorConverter.rgbToXy(msg.red, msg.green, msg.blue, msg.modelidB);\nnode.warn(xy);\nnode.warn(\"RGB output if the RGB matches red and green\");\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n if ((msg.red == rgb.r) && (msg.green == rgb.g)) {\n node.warn(rgb);\n }\n}\nnode.warn(\"Gamut C XY result\");\nxy = ColorConverter.rgbToXy(msg.red, msg.green, msg.blue, msg.modelidC);\nnode.warn(xy);\nnode.warn(\"RGB output if the RGB matches red and green\");\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n if ((msg.red == rgb.r) && (msg.green == rgb.g)) {\n node.warn(rgb);\n }\n}\nnode.warn(\"Gamut D XY result\");\nxy = ColorConverter.rgbToXy(msg.red, msg.green, msg.blue, msg.modelidD);\nnode.warn(xy);\nnode.warn(\"RGB output if the RGB matches red and green\");\nfor (let index = 1; index < 101; index++) {\n rgb = ColorConverter.xyBriToRgb(xy.x, xy.y, index);\n if ((msg.red == rgb.r) && (msg.green == rgb.g)) {\n node.warn(rgb);\n }\n}\nreturn msg;","outputs":1,"noerr":0,"initialize":"","finalize":"","libs":[],"x":1930,"y":500,"wires":[[]]},{"id":"b65a023af1a2f2b8","type":"inject","z":"f1716bd4caacceb3","g":"e66acae9d3caa61c","name":"Input (red, green, blue, light.modelid)","props":[{"p":"payload"},{"p":"red","v":"255","vt":"str"},{"p":"green","v":"228","vt":"str"},{"p":"blue","v":"68","vt":"str"},{"p":"modelidA","v":"LST001","vt":"str"},{"p":"modelidB","v":"LCT001","vt":"str"},{"p":"modelidC","v":"LCT010 ","vt":"str"},{"p":"modelidD","v":"null","vt":"str"}],"repeat":"","crontab":"","once":false,"onceDelay":0.1,"topic":"","payloadType":"date","x":1670,"y":500,"wires":[["8c436eb521dba370"]]}]